Description
You can run your own code before or after data export/import job is executed.
How to enable the feature
- Create a new C# class (let's say ExportImportJobListener, the name does not matter) under Editor assembly (under Editor folder)
- Add the following code to the top of your class
using BansheeGz.BGDatabase.Editor;
- Implement BGEditorJobStartedCallbackI interface if you want to run the code when a job is started
- Implement BGEditorJobCompletedCallbackI interface if you want to run the code when a job is completed
- Context object, which is passed to the methods, provides additional information about operation: the job's name, operation type (import or export) and for BGEditorJobCompletedCallbackI method, the context also has an error (if there was any error)
- See the example below for more details
Example
The following script is executed when a job is started or completed. If you want to share some data between OnStarted and OnCompleted methods, use static fields, because there could be 2 different instances of ExportImportJobListener, one for each interface
using BansheeGz.BGDatabase.Editor;
using UnityEngine;
public class ExportImportJobListener : BGEditorJobStartedCallbackI, BGEditorJobCompletedCallbackI
{
//BGEditorJobStartedCallbackI, called when a job is started (before execution)
public void OnStarted(BGEditorJobStatusContext context)
{
Debug.Log($"Job [{context.JobName}] is started, operation [{context.Operation}]");
}
//BGEditorJobCompletedCallbackI, called when a job is finished
public void OnCompleted(BGEditorJobCompletedContext context)
{
if (context.Error == null)
{
Debug.Log($"Job [{context.JobName}] is completed successfully, operation [{context.Operation}]");
}
else
{
Debug.Log($"Job [{context.JobName}] failed, the error is [{context.Error}]");
}
}
}
Execution result
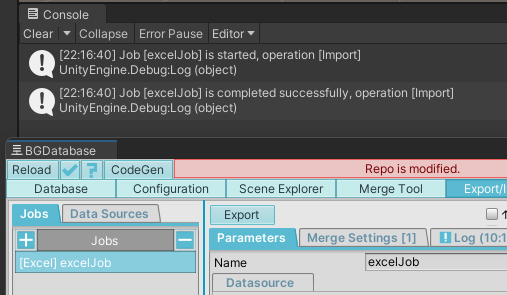